In the realm of web development, the battle between closures and frontal frameworks rages on, each championing its own strengths and weaknesses. This article delves into the intricacies of these two programming paradigms, empowering you with the knowledge to make an informed choice for your next project.
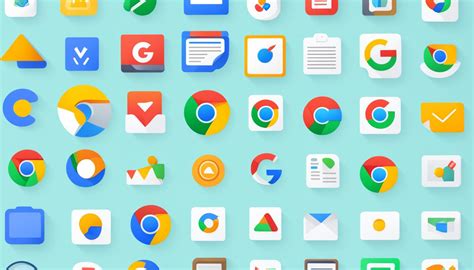
Understanding Closures
Definition: A closure is a function that retains access to the variables of its enclosing scope, even after the function has been returned. This feature enables closures to encapsulate state and behavior in a self-contained unit.
Benefits of Closures:
- Encapsulation: Closures provide a way to hide implementation details and prevent their pollution into the global namespace.
- State Management: Closures can be used to store and manipulate local variables, making state management a breeze.
- Partial Function Application: Closures can be partially applied, allowing you to create specialized functions that can be reused in different contexts.
Example:
// Define a function that returns a closure
function createCounter() {
let count = 0; // Local variable
// Closure function that increments and returns count
return function() {
return count++;
};
}
// Create a counter instance
const counter = createCounter();
// Use the counter instance multiple times
console.log(counter()); // 0
console.log(counter()); // 1
console.log(counter()); // 2
Exploring Frontal Frameworks
Definition: Frontal frameworks are a collection of JavaScript libraries that provide a comprehensive set of tools for building modern web applications. They typically include features such as routing, data binding, and dependency injection.
Benefits of Frontal Frameworks:
- Rapid Development: Frontal frameworks accelerate development by providing pre-built components and code generation tools.
- Consistent UX: Frameworks enforce best practices and conventions, ensuring a consistent user experience across applications.
- Tight Integration: Frontal frameworks often integrate with popular JavaScript libraries and tools, making development seamless.
Example:
// Using Angular, a popular frontal framework
import { Component } from '@angular/core';
@Component({
selector: 'my-component',
template: 'Hello, world!
'
})
export class MyComponent {}
Comparison of Closures vs Frontal Frameworks
Feature | Closures | Frontal Frameworks |
---|---|---|
Encapsulation | Yes | Yes |
State Management | Yes | Yes, but more structured |
Partial Function Application | Yes | Not directly supported |
Code Reusability | High | High (through components) |
Learning Curve | Relatively low | Medium to high |
Performance | Can be less performant | Typically more performant |
When to Use Closures:
- When you need to encapsulate state and behavior within a function.
- When you want to create partial functions that can be reused in different contexts.
When to Use Frontal Frameworks:
- When you need to rapidly develop a modern web application.
- When you want to enforce consistent UX and maintainability.
- When you require deep integration with popular JavaScript libraries and tools.
Creative New Word for New Applications: “Flosure”
To spark innovation, let’s coin a new word: “Flosure.” A Flosure is a hybrid approach that combines the power of closures with the benefits of frontal frameworks. Imagine a world where you can enjoy the flexibility of closures while leveraging the rapid development and structured code of frontal frameworks.
Useful Tables
Table 1: Popular Closures in JavaScript
Closure Type | Use Case |
---|---|
Function Expression | Create functions on the fly |
Anonymous Function | Hide identity and prevent global scope pollution |
Arrow Function | Concise and ES6-compliant |
Table 2: Top Frontal JavaScript Frameworks
Framework | Popularity | Features |
---|---|---|
React | Most popular | Virtual DOM, functional programming |
Angular | Enterprise-grade | Declarative templates, dependency injection |
Vue.js | User-friendly | Progressive, reactive framework |
Table 3: Questions to Ask Your Customers
- What are their key requirements for the application?
- What is their preferred development workflow?
- What existing JavaScript libraries and tools do they use?
Table 4: Tips and Tricks for Using Closures and Frontal Frameworks
- Use closures sparingly: Avoid excessive use as they can impact performance.
- Name your closures clearly: This helps with readability and maintainability.
- Leverage framework features: Explore built-in tools to streamline development.
- Test your code thoroughly: Ensure functionality and prevent unexpected behavior.
Conclusion
Understanding the nuances of closures and frontal frameworks empowers you to make informed choices for your web development projects. By considering the advantages and disadvantages of each approach, you can harness their unique strengths to create powerful and efficient applications. Remember, fostering open communication with your customers is crucial to align your solutions with their specific needs.