Understanding Closure
A closure is a function that has access to the variables in the lexical scope even when the function is invoked outside of that lexical scope. Closures are frequently used in JavaScript and other programming languages to create private variables and methods, implement higher-order functions, and simulate class-like behavior.
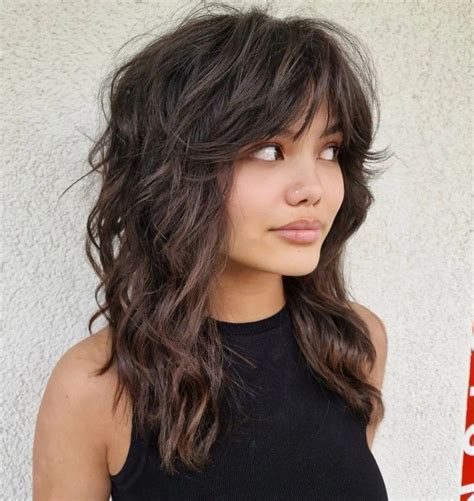
Benefits of Closure
- Encapsulation: Closures allow for the creation of private variables and methods, enabling encapsulation and data hiding.
- Higher-order functions: Closures can accept other functions as arguments and return functions as values, making it possible to implement higher-order functions that operate on other functions.
- Event handling: Closures can be used to define event handlers that have access to specific data, simplifying event handling and reducing code duplication.
Common Mistakes to Avoid
- Overusing closures: While closures are powerful, overuse can lead to memory leaks and performance issues. Avoid creating unnecessary closures and release them when no longer needed.
- Capturing large objects: Capturing large objects within closures can lead to increased memory usage and slow down performance. Consider using weak references or other techniques to manage memory efficiently.
- Dangling references: Closures can hold references to variables that have gone out of scope, leading to dangling references. Ensure that closures only reference variables that are still in scope.
Understanding Frontal
Frontal is a software design pattern that defines a single point of entry for a software system. The frontal component is responsible for receiving all requests and delegating them to the appropriate internal components or services.
Benefits of Frontal
- Centralized control: Frontal provides a single point of control for all incoming requests, simplifying system administration and maintenance.
- Security: By centralizing access, frontal can implement security measures and access control mechanisms to protect the internal system.
- Scalability: Frontal can be scaled horizontally by adding additional instances, increasing the overall system’s throughput and capacity.
Common Mistakes to Avoid
- Lack of decoupling: Avoid creating a tight coupling between the frontal component and the internal system. This can make it difficult to maintain, test, and scale the system.
- Overloading the frontal: The frontal component should not be overloaded with too many responsibilities. Keep the frontal lean and focused on its primary function of request handling.
- Ignoring performance: Frontal can become a bottleneck if not properly designed and optimized. Ensure that the frontal component can handle the expected load efficiently.
Comparison of Closure and Frontal
Both closure and frontal are essential tools in software development, but they serve different purposes:
Feature | Closure | Frontal |
---|---|---|
Purpose | Access to lexical scope | Single point of entry |
Scope | Local to a function | Global to the system |
Encapsulation | Private variables and methods | N/A |
Control Flow | Allows deviation from normal flow | Ensures structured flow |
Flexibility | Highly flexible | Less flexible |
Applications of Closure and Frontal
Applications of Closure
- Private data encapsulation: Closures can be used to create private variables and methods, preventing direct access from outside the closure’s scope.
- Higher-order functions: Closures enable the implementation of higher-order functions that operate on other functions, providing greater flexibility and code reusability.
- Event handling: Closures can be used to create event handlers that have access to specific data, reducing code duplication and improving maintainability.
Applications of Frontal
- Request routing: Frontal acts as a central hub, routing incoming requests to the appropriate internal components or services based on request parameters.
- Security: By centralizing access, frontal can implement security measures such as authentication, authorization, and encryption.
- Scalability: Frontal allows for horizontal scaling by adding additional instances, increasing the overall system’s capacity and performance.
Why Closure and Frontal Matter
Closure and Frontal are essential components of modern software development:
- They provide greater control and flexibility over code structure and execution flow.
- They enhance security by encapsulating data and implementing security measures.
- They improve scalability by centralizing request handling and enabling horizontal scaling.
Benefits of Using Closure and Frontal
Organizations that embrace closure and frontal experience significant benefits:
- Increased code quality: By encapsulating data and controlling access, closures and frontal reduce the risk of errors and improve code maintainability.
- Enhanced security: Frontal provides a centralized point for implementing security measures, protecting the system from threats.
- Improved performance: Frontal allows for efficient handling of incoming requests and supports horizontal scaling, ensuring optimal system performance.
Tips and Tricks
Tips for Closure
- Use closures sparingly to avoid memory leaks and performance issues.
- Capture only necessary variables within closures to reduce memory usage.
- Use weak references to avoid dangling references.
Tips for Frontal
- Decouple the frontal component from the internal system to enhance flexibility.
- Avoid overloading the frontal component with excessive responsibilities.
- Optimize the frontal component for performance to handle high request volumes efficiently.
Conclusion
Closure and frontal are two fundamental concepts in software development that provide powerful tools for creating efficient, secure, and scalable applications. By understanding their distinct roles and applications, developers can leverage these tools to enhance code quality, improve security, and maximize system performance.